Unlocking the Power of List Comprehensions in Python
Written on
Chapter 1: Understanding List Comprehensions
In our previous discussion about list comprehensions in Python, we explored their syntax and basic usage. This follow-up article aims to delve deeper into practical applications, particularly in data processing, given the popularity of the initial piece.
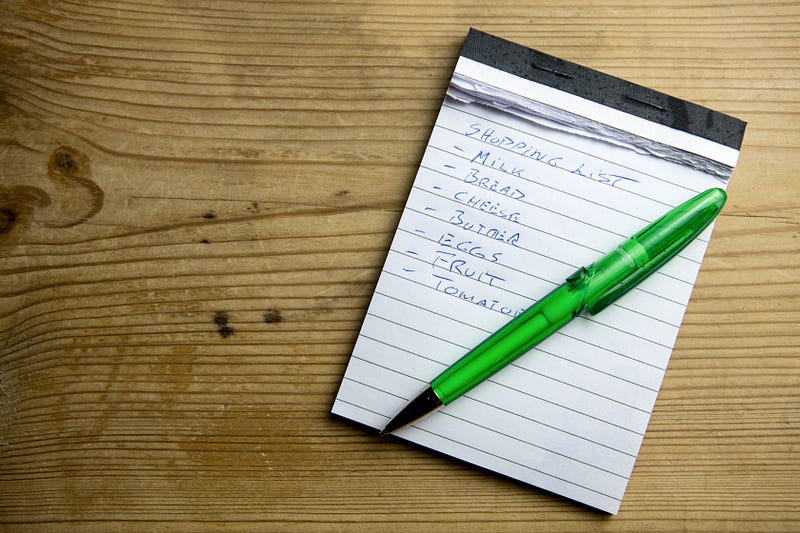
Photo by Torbjørn Helgesen on Unsplash
Section 1.1: A Closer Look at Syntax
Last time, we examined the structure of list comprehensions in detail. Before diving into data examples, let’s clarify a more intricate comprehension. Consider this code snippet that applies a user-defined function to a list of words, but only for those with four or more letters:
def abbreviate_word(word):
return word[0] + word[-1] # Extracts first and last letters
words = ['hi', 'bye', 'sun', 'moon', 'sky', 'earth']
abbrevs = []
for word in words:
if len(word) >= 4:
abbrevs.append(abbreviate_word(word))
Can we streamline this into a list comprehension? Absolutely! Here’s how:
words = ['hi', 'bye', 'sun', 'moon', 'sky', 'earth']
abbrevs = [abbreviate_word(word) for word in words if len(word) >= 4]
This single line encapsulates our logic neatly. The filtering occurs first, ensuring that only words meeting our length criteria are processed, resulting in the list ['mn', 'eh'].
Section 1.2: Applying List Comprehensions in Data Analysis
Now, let’s explore how to utilize list comprehensions in data contexts. A prime example involves employing a basic MapReduce approach. For those unfamiliar, MapReduce refers to the processes of filtering and transforming data, followed by aggregation into a single value. List comprehensions can effectively replicate this model.
While many data programmers rely on Pandas DataFrames, it’s essential to note that data can also be structured as dictionaries. For instance, consider a dataset from MongoDB that maps cities to their recent COVID case numbers:
covid_cases = {
'Minsk': 3000, 'Lahore': 1200, 'Colombo': 800,
... , 'Miami': 8000
}
Your objectives might include calculating the average number of cases in cities with at least 1,000 cases, identifying cities with fewer than 3,000 cases, and computing the sum of squares of all cases. All of these can be efficiently accomplished using list comprehensions:
from numpy import mean
# Average cases
average_cases = mean([value for value in covid_cases.values() if value > 1000])
# Cities with fewer than 3000 cases
cities_below_3000 = [key for (key, value) in covid_cases.items() if value < 3000]
# Sum of squares of cases
sum_of_squares = sum([value * value for value in covid_cases.values()])
These concise operations illustrate the convenience and efficiency of list comprehensions.
Chapter 2: Simulating Experiments with List Comprehensions
In this video, titled "List Comprehension Part 2," we continue our exploration of list comprehensions in Python, focusing on practical applications and examples.
Next, let’s demonstrate how list comprehensions can simplify experimental simulations. For example, consider simulating a coin toss, as referenced in UC Berkeley's introductory data science materials:
import numpy as np
import random
def one_simulated_value():
outcomes = np.random.choice(['Heads', 'Tails'], 100)
return np.count_nonzero(outcomes == 'Heads')
num_reps = 20000 # Number of repetitions
heads = []
for i in range(num_reps):
new_value = one_simulated_value()
heads.append(new_value)
This simulation can be streamlined using a list comprehension:
[heads.append(one_simulated_value()) for i in range(num_reps)]
This approach not only condenses the code but also enhances readability, even when the output is not utilized.
Final Thoughts
Though this article only scratches the surface, it highlights the utility of list comprehensions in Python programming. I hope the detailed examples provided offer you a clearer understanding of how to leverage these comprehensions for data processing. Remember, the goal is to cultivate cleaner and more Pythonic code, a mindset that will pave the way for your success.
Until next time!
In this video, "Python Crash Course: Part 11 - Using List Comprehensions," we further explore the practical applications of list comprehensions in Python.