Mastering Essential Spring Annotations for REST API Development
Written on
Understanding the Core Annotations
To effectively work with Spring REST APIs, it's essential to have a proper setup, including a Java IDE like IntelliJ and an API testing tool such as Postman. Additionally, ensure you have a basic Spring REST API project created using Spring Initializr.
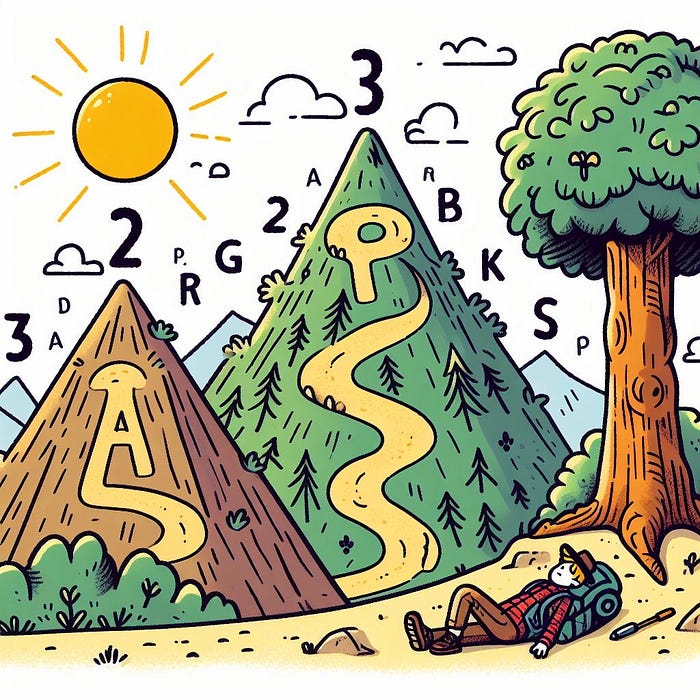
Annotation #1: @RestController
The @RestController annotation transforms a class into a REST controller, allowing it to manage HTTP requests and generate responses, typically in formats like JSON or XML. While powerful, @RestController works best when paired with other annotations, such as @GetMapping.
For instance, the following code snippet demonstrates a simple API endpoint:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class AppointmentAPI {
@GetMapping("/appointments/hello")
public String hello() {
return "hello from AppointmentAPI!";}
}
Refer to the video linked below for a detailed demonstration of creating and testing this API.
Annotation #2: @RequestMapping
When dealing with multiple endpoints, specifying the complete path for each can be cumbersome. The @RequestMapping annotation allows you to set a base path for all methods in a class, simplifying your endpoint definitions.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/appointments")
public class AppointmentAPI {
@GetMapping("/hello")
public String hello() {
return "hello from AppointmentAPI!";}
}
Refer to the video linked below for a detailed demonstration of creating and testing this API.
Annotation #3: @GetMapping and #4: @PathVariable
The @GetMapping annotation is commonly used to retrieve data, such as listing or viewing details. Below is an example of how to implement these functionalities:
@GetMapping("/")
public List<AppointmentDTO> list() {
return this.appointments;
}
@GetMapping("/{id}")
public AppointmentDTO view(@PathVariable("id") Long id) {
return this.appointments.stream()
.filter(a -> a.id.equals(id))
.findFirst()
.orElse(null);
}
Refer to the video linked below for a detailed demonstration of creating and testing this API.
Annotation #5: @PostMapping and #6: @RequestBody
The @PostMapping annotation is utilized to handle HTTP POST requests, allowing you to create new entities such as appointments. The @RequestBody annotation enables automatic extraction of data from the request body.
@PostMapping("/")
public void create(@RequestBody AppointmentDTO appointmentDTO) {
appointmentDTO.id = lastId++;
this.appointments.add(appointmentDTO);
}
Refer to the video linked below for a detailed demonstration of creating and testing this API.
Annotation #7: @PutMapping
The @PutMapping annotation is used for updating existing entities. Typically, you will include an ID in the endpoint to identify the entity being updated.
@PutMapping("/{id}")
public void update(@PathVariable("id") Long id, @RequestBody AppointmentDTO appointmentDTO) {
var existingAppointment = this.appointments.stream()
.filter(a -> a.id.equals(id))
.findFirst()
.orElse(null);
if (existingAppointment != null) {
existingAppointment.title = appointmentDTO.title;
existingAppointment.date = appointmentDTO.date;
}
}
Annotation #8: @DeleteMapping
The @DeleteMapping annotation is used to remove an existing entity, such as canceling an appointment. It generally requires only an ID as a path variable.
@DeleteMapping("/{id}")
public void delete(@PathVariable("id") Long id) {
var existingAppointment = this.appointments.stream()
.filter(a -> a.id.equals(id))
.findFirst()
.orElse(null);
if (existingAppointment != null) {
this.appointments.remove(existingAppointment);}
}
Annotation #9: @RequestParam
Finally, the @RequestParam annotation is valuable for handling search queries within your API.
@GetMapping("/search")
public List<AppointmentDTO> search(@RequestParam("date") String date) {
return this.appointments.stream()
.filter(a -> a.date.equals(LocalDate.parse(date)))
.collect(Collectors.toList());
}
Summary
This article has introduced nine fundamental annotations that are critical for enhancing your REST API expertise in Spring. Mastering these annotations will significantly improve your coding capabilities in RESTful services. In future articles, we will delve deeper into specific annotations, exploring advanced use cases and expert techniques. Feel free to reference the provided sample codes or attempt to create them on your own.